Adding healthchecks to your APIs is generally a good idea as it can provide some useful information about the status of your services. There’s a lot of good guides about how to do this including the offical Microsoft documentation but there’s fewer guides on how to set up your own custom healthchecks and how to have the healthchecks running on a separate port to the main application.
An example project demonstrating the below features can be found here.
Custom Healthchecks
Custom healthchecks can be used to check the status of external resources such as 3rd party APIs, these are written to against the interface IHealthCheck
.
public class IpRegistryHealthCheck : IHealthCheck
{
private readonly IIpRegistryService _ipRegistryService;
public IpRegistryHealthCheck(IIpRegistryService ipRegistryService)
{
_ipRegistryService = ipRegistryService;
}
public async Task<HealthCheckResult> CheckHealthAsync(HealthCheckContext context, CancellationToken cancellationToken = default)
{
try
{
var response = await _ipRegistryService.GetIpAddressDetailsAsync();
if (response != null)
{
return HealthCheckResult.Healthy("IP Registry API available");
}
else
{
return HealthCheckResult.Unhealthy("IP Registry API not returning data");
}
}
catch (Exception ex)
{
return HealthCheckResult.Unhealthy("IP Registry API connection failed");
}
}
}
These can then be added on startup.
services.AddHealthChecks()
.AddCheck<MetOfficeDataPointHealthCheck>("MetOfficeDataPoint")
.AddCheck<IpRegistryHealthCheck>("IpStack")
.ForwardToPrometheus();
Setting the Healthcheck Port
If you want the healthcheck endpoint to run on a different port than your main application then this needs to be specified when you map your healthchecks to a specific endpoint.
app.MapHealthChecks("/healthcheck", new HealthCheckOptions
{
ResponseWriter = WriteResponse
}).RequireHost("*:8880");
In this case the healthchecks are output on port 8880, however by default this port isn’t open on the server so this needs to be configured in the WebHost
.
builder.WebHost.ConfigureKestrel(options =>
{
options.Listen(IPAddress.Any, 8880, listenOptions =>
{
listenOptions.Protocols = HttpProtocols.Http1AndHttp2;
listenOptions.UseHttps();
});
options.Listen(IPAddress.Any, 7183, listenOptions =>
{
listenOptions.Protocols = HttpProtocols.Http1AndHttp2;
listenOptions.UseHttps();
});
options.Listen(IPAddress.Any, 5183, listenOptions =>
{
listenOptions.Protocols = HttpProtocols.Http1AndHttp2;
});
});
The other ports are already defined in launchSettings.json and can be changed here if required.
"DockerTest": {
"commandName": "Project",
"launchBrowser": true,
"launchUrl": "swagger",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
},
"applicationUrl": "https://localhost:7183;http://localhost:5183",
"dotnetRunMessages": true
}
By default Visual Studio runs the web application in IIS if you just hit F5, in order for it to use the above ports you need to run the site directly. This can be done by changing the dropdown or by using the dotnet run
command.
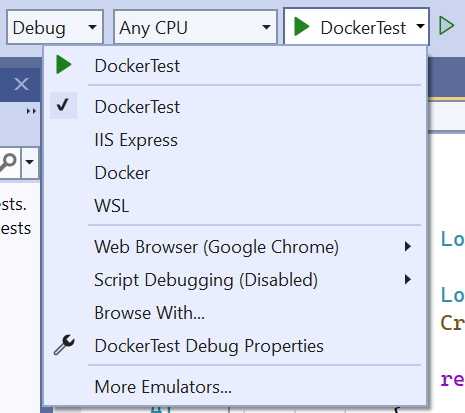
0 Comments