I’ve started to learning about Docker and as part of that process I’ve initially been trying to just deploy a simple test API to a locally running Docker container.
In order to get a basic proof-of-concept site up and running I first created a new ASP .NET Core Web API project in Visual Studio.
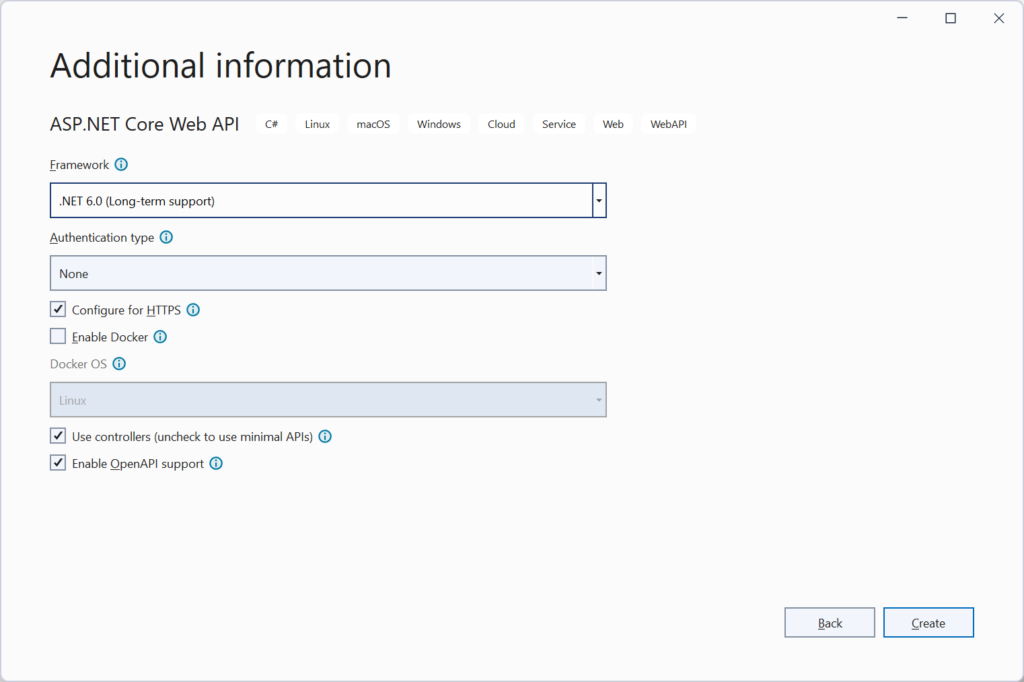
Once the project has been created you can either get your site working in Docker by using Visual Studio to add all the required files using automatically or you can add them yourself.
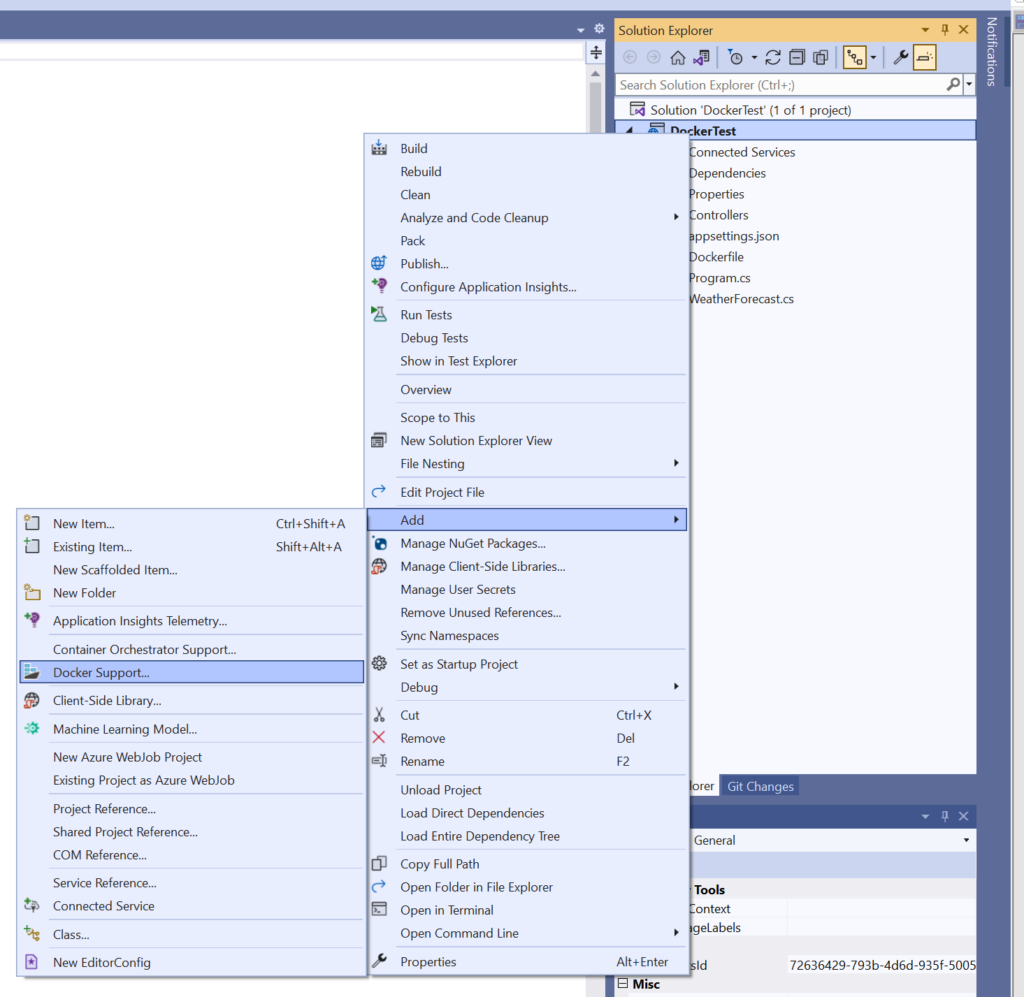
If you use Visual Studio to setup Docker for you then it will run in it’s own internal Docker instance and should just magically work.
If you want to get it working manually then you will first need to install Docker Desktop and then add a Dockerfile to your project.
FROM mcr.microsoft.com/dotnet/aspnet:6.0 AS base
WORKDIR /app
EXPOSE 80
EXPOSE 443
FROM mcr.microsoft.com/dotnet/sdk:6.0 AS build-env
WORKDIR /app
# Copy everything
COPY . ./
# Restore as distinct layers
RUN dotnet restore
# Build and publish a release
RUN dotnet publish -c Release -o out
# Build runtime image
FROM mcr.microsoft.com/dotnet/aspnet:6.0
WORKDIR /app
COPY --from=build-env /app/out .
ENTRYPOINT ["dotnet", "DockerTest.dll"]
In the above code DockerTest is the name of my project so this will need to change if the project is called something else.
Once that’s done you can build a Docker image with the name of dockertest using the below command.
docker build -t dockertest .
To run this image you can use the following command, in it you need to specify the name of your image as well as the name of the container you’re running it in (if this doesn’t exist it will be created).
docker run -d -p 8000:80 --name dockertestcontainer dockertest
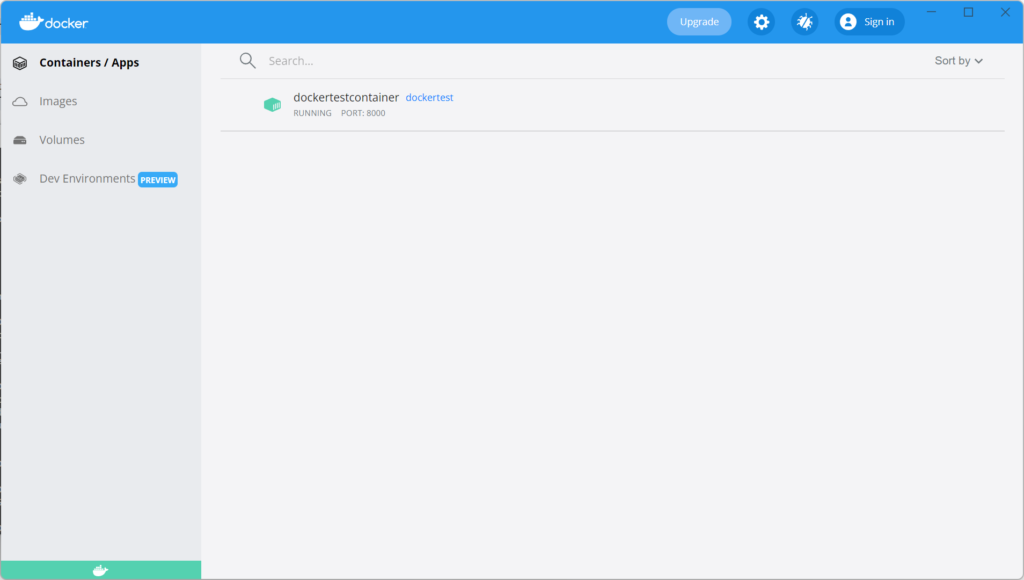
Once your image is running you can test it by hitting http://localhost:8001/weatherforecast. When running locally in Visual Studio debug the root path redirects to Swagger but in Docker this is not the case and instead you recieve a 404. This is because in the template Swagger is only enabled in debug enviroments and the Docker image is built in the release enviroment.
If the site is running in Visual Studio then both HTTP and HTTPS routes will be accessable, however in Docker the HTTPS route has to be explicitly specified and a certificate file has to be loaded as described here.
First off you need to export the .NET development certificate to a file location, this certificate has to be password protected (though ideally with a better password than “password” used below).
dotnet dev-certs https -v -ep C:\TEMP\cert-aspnetcore.pfx -p password
Once done you can then specify the ports used for HTTP (80) and HTTPS (443) traffic, map the rewrites and tell the kestral server where the certificate is.
docker run -d -p 8000:80 -p 8001:443 -e ASPNETCORE_HTTPS_PORT=8001 -e ASPNETCORE_URLS=https://+;http://+ -e Kestrel__Certificates__Default__Path=/root/.dotnet/https/cert-aspnetcore.pfx -e Kestrel__Certificates__Default__Password=password -v C:\TEMP\:/root/.dotnet/https --name dockertestcontainer dockertest
This will launch a container with both HTTP and HTTPS endpoints and HTTP > HTTPS rewrites enabled.
0 Comments